Introduction
Photoshop provides a number of tools that
aid in automating many tasks involved in image editing. This article
provides an introduction to
one of the more powerful tools at your disposal: scripting. A couple
of examples are presented as is a list of resources that will help
should you decide that scripting should become a part of your Photoshop
toolkit.
An Overview of Scripting in Photoshop
A script, at its most basic,
is a text file containing a set of commands. Batch/Command files
in WinXP and shell scripts in Unix-based systems
are both examples of scripts that most people are familiar with.
In the case of Photoshop, the commands that are available make
it possible to manipulate image files.
If there was an English language
interpreter in Photoshop, the commands might look something like:
Rotate the current document 90° clockwise.
Flatten the layers.
Save the document as a web jpeg file at quality level 8.
As readable as this may be, the ambiguity inherent to
the English language makes it a bad choice for scripting. Fortunately,
Adobe provides three other languages (AppleScript, JavaScript,
and VBScript) for scripting Photoshop.
The next two sections
discuss when scripting is appropriate (compared to other techniques)
and what factors you
need to consider when
deciding what the best language will be for your scripting tasks.
When is Scripting Appropriate
When faced with a repetitive task in
Photoshop, there are a number of different ways to simplify the task.
Some solutions are more difficult
than other, but they all have a place. However it is important
to remember that scripting is superset of the Action and Batch functionality
already present in Photoshop. Anything you can do with Batch and
Actions you can also do in a script. Additionally, scripting puts
a wider array of tools and techniques at your disposal.
This is
a useful checklist to go through when trying to optimize a repetitive
task. This first solution is easiest, the last is
the most
complex.
1.Do it manually. This is the simplest solution. It's appropriate
when you only have to do something a few times and the cost
of finding
or implementing another
solution is higher than the cost of just getting the task done.
2. Use an automation plugin. There are several automation plugins
that come with Photoshop (under the File->Automate menu) that
may take care of the problem. There are also a wide variety of
3rd party plugins
available online. One of these
may be what you need.
3. Use an Action. Photoshop provides some useful Actions and there
are many thousands of free and commercial Action sets available
on web
sites all
over the Internet.
4. Use an existing script. You can find a lot bundled with Photoshop
(under the File->Scripts menu) , on Adobe's site, and elsewhere
on the web.
5. Record an Action. Many times the problem you are trying to solve
is very specific to your workflow or to some set of images
that you're working
on. Existing
Actions or plugins may not do what you need. The ability to
record, replay, and batch
Actions let's you develop a set of automation tools that address
your exact set of needs.
6. Write a script. Although quite a bit more complex than recording
an Action, this technique is also considerably more powerful.
Anything you can do
in an Action
can be done in a script. There is at least one Action file
to JavaScript translator [see Resources] that illustrates
this fact.
But, in
addition to all of capabilities
of Actions, you also now have the ability to branch and iterate
automatically. Examples of this will appear in the next section.
7. Write an automation plugin. While this is the most complex
solution it is, ultimately, the most powerful. Writing
in compiled programming
language
(i.e.
C++) gives
you far fuller access to Photoshop's runtime environment
and makes it possible to implement highly complex solutions
with
good performance.
In the end,
it is not uncommon that a highly automated workflow uses some combination
of Actions, scripts, and plugins to accomplish the required
tasks. Understanding
what the available tools are capable of and where there are appropriate
goes a long way towards this end.
Selecting the Appropriate Scripting Language
Photoshop can be scripted
from a number of different languages. Specifically, these languages
are:
- JavaScript (JS) – Available for both Windows and Macintosh
computers. It provides a standard core JavaScript implementation
with extensions for programming
Photoshop. This has nothing to do with the Java language except having
a similar name. Also, this JavaScript interpreter has nothing to
do
with web browsers,
although it can be used to generate HTML pages.
- AppleScript (AS)– Available
only on the Macintosh.
- VBScript (VBS) – Available only on Windows.
- OLE interoperable
languages – Available only on Windows.
Includes VisualBasic, C#, and other languages. This is only really
an appropriate
choice if you
have a good background in OLE programming and you have an existing
body of code that
you plan on utilizing.
How you select a language for scripting depends on
your needs, your experience, and your platform. Here are a set of relevant
questions that you should
ask yourself when selecting a language.
1. On what platform do the scripts have to run? On Windows, you
have a choice between VB and JS, on the Macintosh you have a choice
between
JS and
AS. On both Windows
and Macintosh computers? If so, your only choice is JS.
2. Does
your script have to work with other Creative Suite applications?
JS provides the best support although AS and VBS can be used to
some extent
on their respective
platforms.
3. Does your script have to work with other non-Creative Suite applications?
This is the reverse of the previous item. AS and VBS are likely
going to be better
suited although there will be situations where JS will work
just fine.
4. Do you have a background in writing scripts? If so, one of
the languages is likely more a appropriate choice for you.
5. What does everybody else do? There are a lot of people out
there writing scripts for Photoshop. If you are interested
in leveraging
scripts
that they've written
or interacting with them on available online forums, choosing
the same language might be important. A rough estimate
would place JS at around
90% of the available
3rd party scripts and 85% of the forum traffic on various
sites.
You
may have noticed that this discussion mentioned nothing about language
details. JS, AS, and VBS all have similar capabilities at the language
level. The factors
listed above will likely have more bearing on your choice of a scripting
language than specific details about syntax.
A Simple Example
For a simple, but useful, example, let's look at a
common requirement. We have an Action, 'Wood Frame #2' in Action
set 'Wood Frame', that
does what we need. However, it only works on landscape images and
we have a mix of landscape and portrait ones. What we need to do
is:
- If the image is in portrait mode, rotate the image 90° clockwise,
run the Action, and rotate the image back.
- If the image is in
landscape mode, just run the Action.
Here is what the JavaScript
for this would look like:
doc = activeDocument;
if (doc.height > doc.width) {
doc.rotateCanvas(90);
doAction('Wood Frame #2', 'Wood Frames');
doc.rotateCanvas(-90);
} else {
doAction('Wood Frame #2', 'Wood Frames');
}
Now, to explain this in detail.
- The first line doc = activeDocument says that we want to use 'doc'
to refer to the top-most document in Photoshop.
- The line if
(doc.height > doc.width) { says that if the
height is greater than the width (portrait mode), execute the
next three lines. If not, execute
the line after the } else {.
- The doc.rotateCanvas(90)/doc.rotateCanvas(-90) lines rotate
the image clockwise and counter-clockwise respectively.
- The
doAction('Wood Frame #2', 'Wood Frames') lines do the work of running
the Action from the Actions Palette.
The Photoshop JavaScript
interpreter executes these lines of code in order and branches,
if necessary, on the 'if' statement.
Now that we have this script, we
can modify it to work with other Actions by simply changing the names
of the Action and Action set
in the script.
To try
this out, save this to a text file. Replace the Action with 'Image
Effects – Blizzard'
that comes with the PSCS2 distribution. If you then run the script
(from File->Scripts->Browse),
you'll see portrait images rotate before the Action is executed.
As a result, the 'snow' is going in a different direction than
it is in landscape images.
Whether this example is easy or not
depends much on your technical background. If you've had any
exposure to programming languages,
it shouldn't be
too hard to get a handle on what's going on in this script.
If programming languages are something you're not familiar with,
it may take a bit
more work to get
comfortable
with some of the concepts. The References section has pointers
to books and websites that provide good help in learning the
JavaScript language.
A Complex Example
For a complex example, we will look at the Image
Processor script that comes with Photoshop CS2. We will be looking
at what scripting
is capable of. A detailed look at the code is well beyond the
scope of this article.
The Image Processor script is a kind of batch processor. With
it, you can select source and destination folders, the file
types and
sizes to save, an optional Action to run, along with a few other
properties. You can save your configuration for a batch run to
a preferences file.
The script can be launched from File->Scripts->Image
Processor. The script itself is located at PS Directory/Presets/Scripts/Image
Processor.jsx.
When you run the Image Processor script, you are
presented with this window:
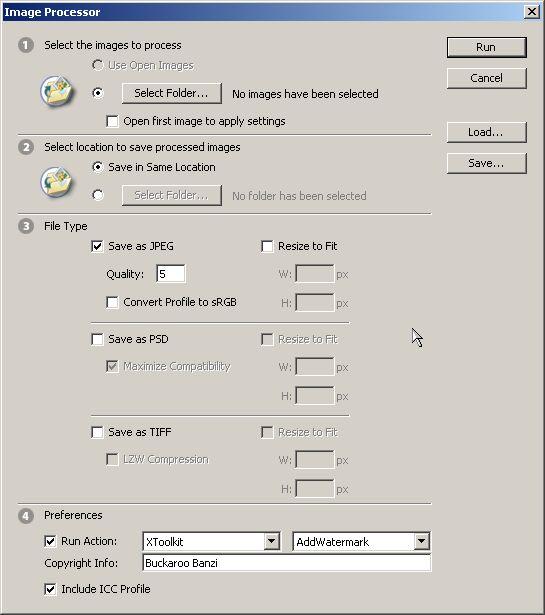
This script obviously could not have been implemented
by using the standard Batch and Action facilities of PSCS2.
- Sections 1 and 2 look similar to panels in the standard
Batch window, except for the 'Open first image' check box which
facilitates work
with sets of RAW
images.
- Batch does not have the capability to load and save batch run preferences.
- In
Section 3, you have the ability to choose file types and dimensions
for a batch run. The best you can do without scripting is to
record resize and
saveAs
steps for all three file types into an Action. Before running the batch
you would have to manually change the Action to specify which
resize and saveAs
steps are
active. This can be a very error prone exercise.
- In Section 4, as in
Section 3, these fields could all become steps in an Action but
would require the same tedious modification of the
Action
before each batch
run.
The programming power of the scripting language and Photoshop libraries
makes it possible to write sophisticated scripts with a slick user
interface and
broad functionality.
In reality, most scripts that people write for
Photoshop are not this complex and many have no user interface at
all.
Resources
Books and Documentation
All of Adobe's documentation for scripting
Photoshop can be downloaded from here. The Scripting Guide gives
a good overview of how scripting
fits into Photoshop. The Reference Guides are essential for finding
out how to do anything at all with the scripting APIs. All of these
PDFs are included with the PSCS2 distribution.
For
a more introductory level book, I've heard good things about
Beginning JavaScript (Wilton), but there are a great
many other
books on the
market for getting started with JavaScript. Check your
local bookstore.
For an online JavaScript reference
www.croczilla.com is a fairly good site. There are others out there
as
well.
Google can point
you to them
if croczilla doesn't work for you.
Tutorials
kirupa.com has probably the best (only) online tutorials
for using JavaScript with Photoshop. Highly recommended.
User Forums
The Photoshop Scripting Forum hosted by Adobe provides
access to some of the best and brightest Photoshop scripting minds
in and outside
of Adobe. The volume is around six to ten posts a day and the signal
to noise ratio is good.
www.ps-scripts.com was
setup last year by Andrew Hall and others as a compliment to the Adobe
Forum. In addition
to handling a bit
more
traffic than the Adobe Forum, ps-scripts also hosts scripts that
have been uploaded by members of the community as well as provides
a place
for people to get basic support for those scripts.
If you are going
to be doing any more than basic scripting with Photoshop, you'll
find yourself visiting these two sites on a regular
basis. Scripts and Other Related Stuff
In addition to the scripts that come
with PSCS2, you can visit Adobe Studio Exchange and find around sixty
scripts that have been uploaded
by other Photoshop scriptwriters. There's a lot of good stuff there.
Scripts are also available at www.ps-scripts.com and other sites
around the Internet.
One last site that needs mentioning is atncentral.com.
They have the best online Actions tutorial as well as a nice collection
of
free Actions
available for download. It's an excellent resource. Conclusion
Hopefully this article helped to shed some light on the
What, How, and Why of scripting in Photoshop. The Resources section
contains
an excellent set references to information that will prove helpful
should you decide take advantage of the scripting capabilities
in Photoshop.
About the Author
I have a long history in software engineering and
photography. Scripting Photoshop has turned out to be excellent way
to engage both of these
passions. In addition to providing support to other scriptwriters
on the Adobe Scripting Forum and ps-scripts.com, I also offer online
consulting services for those in need of additional help.
You can contact xbytor here. |